The community is working on translating this tutorial into Polish, but it seems that no one has started the translation process for this article yet. If you can help us, then please click "More info".
View Discovery: Connecting Controller & View
In the beginning of this tutorial, I demonstrated how a Controller can return a View simply by calling the View() method. This even works without parameters, so at that point, you might wonder how in the world a Controller knows exactly which of your Views to return for a specific Action. The answer is something called View Discovery - a process where ASP.NET MVC will try to guess which View to use, without forcing you to specify it.
View Discovery works when you follow a certain convention when creating your project structure. We have already talked about this previously in this tutorial, where we always put Controllers in a folder called "Controllers", Models in a folder called "Models" and so on. With Views, you should place them inside a sub-folder named after the Controller and the filename of the View should match the name of the Action. So for instance, if you have a controller called "ProductController", with an action called "Details", your folder structure should look like this:
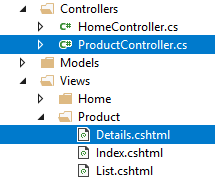
In other words, the location and naming of your views should follow this convention:
/Views/[Controller Name]/[Action Name].cshtml
If it does, you can simply call the View() method from your Controller actions and have the .NET framework automatically locate the proper View for you:
public class ProductController : Controller
{
public IActionResult Index()
{
return View();
}
public IActionResult Details()
{
return View();
}
}
If the framework can't find a matching View using the convention mentioned above, it will look in one more place:
/Views/Shared/[Action Name].cshtml
The Shared folder, which you may add inside your Views folder, is usually used for Layout files and partial views shared across multiple controllers. We'll discuss that later on, but for now, the easiest thing is to follow the folder structure convention outlined above.
Specifying a View
If you can't (or won't) follow the convention, you can help the framework locating the matching View by supplying a parameter to the View() method. There are two ways: You can either specify just the name, which still requires you to follow the folder-structure-convention, but supply a View with another name (think of it as an alias) like this:
public IActionResult Test()
{
return View("Details");
}
Or you can supply the entire path, which gives you complete flexibility when creating your project structure:
public IActionResult Test()
{
return View("/ViewFolderName/SomeFolderName/ViewName.cshtml");
}
Summary
If you follow a specific convention when creating your project structure, View Discovery allows your Controllers to automatically find a matching View based on the name of the Controller and the Action. If you don't follow this convention, you can easily return a specific View by passing it's path and/or name to the View() method.